Installing the SDK
The Paragon SDK can be imported in your front-end JavaScript to embed the Connect Portal and trigger workflows in your application.
Installing from npm
You can install the Paragon SDK with npm:
The SDK can be imported in your client-side JavaScript files as a module:
For on-premise/single-tenant users:
If you are using an on-prem/single-tenant instance of Paragon, you can call the .configureGlobal
function to point the SDK to use the base hostname of your Paragon instance.
Setup
Before using the Paragon SDK, you’ll need to set up your application to verify the identity of your users to the SDK.
Paragon verifies the identity of your users using the authentication system you’re already using, including managed services like Firebase or Auth0. Some backend code may be required if your application implements its own sign-in and registration.
Generating tokens with your backend
If your backend server will generate JWTs for Paragon, you’ll first need to complete the following steps:
1. Generate a Paragon Signing Key
To generate a Signing Key, go to Settings > SDK Setup in your Paragon dashboard. You should store this key in an environment secrets file. For security reasons, we don’t store your Private Key and cannot show it to you again, so we recommend you download the key and store it someplace secure.
A unique Signing Key is generated for each environment. To learn more about the environments supported by Paragon, visit our Releases documentation.
Note: Signing keys never expire. However, generating a new signing key for the same environment will automatically invalidate the previous one.
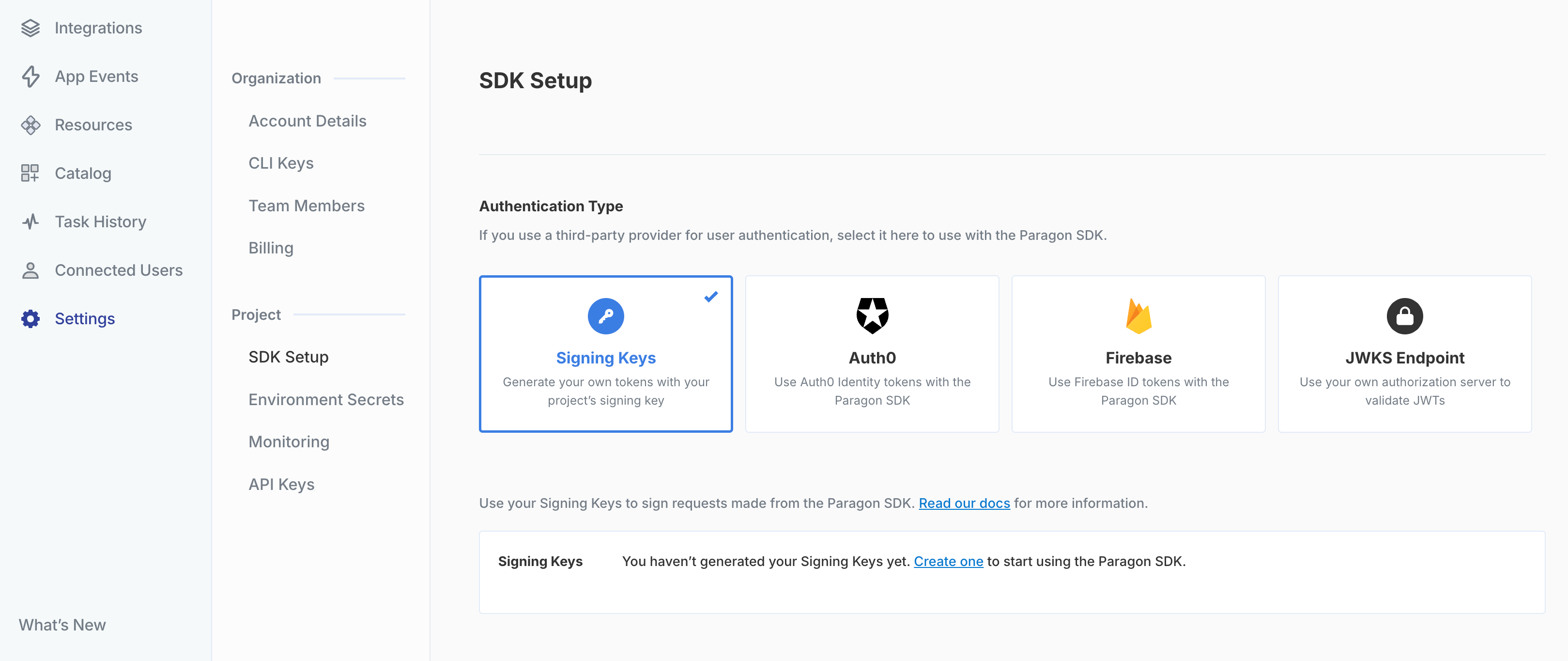
2. Generate a Paragon User Token
Next, you’ll need to generate a Paragon User Token for each of your authenticated users. To do this, you’ll need a library in your target language to sign JWTs with RS256. You can find one in your language at https://jwt.io/.
If your application is a fully client-rendered single-page app, you may have to create and use an additional API endpoint to retrieve a signed JWT (or reuse an existing endpoint that handles authentication or user info).
The signed JWT/Paragon User Token minimally must include the sub
, iat
, and exp
claims:
Just for testing: Generating one-off JWTs
Use the Paragon JWT Generator to generate test JWTs for your development purposes. In production, static tokens should never be used.
3. Call paragon.authenticate()
You’ll call paragon.authenticate
in your view with a JWT signed by your backend using the library chosen in Step 2. This JWT is the Paragon User Token.
The paragon.authenticate
function is Promiseable and resolves when the SDK has successfully authenticated your user. Note that other functions, like paragon.connect
, may not work as expected until this Promise has resolved.
Example Implementation: A Node.js and Express app using Handlebars view templating
-
Adding middleware to sign the JWT and include it in the response context
-
Use the
paragonJwt
set in context within the view template, with a call toparagon.authenticate
:
Using a managed authentication service
If you already use an authentication service like Auth0, Firebase, or a JWKS-compatible authentication server, you can set up your Paragon project to use tokens generated by that service by navigating to Settings > SDK Setup and selecting a provider.
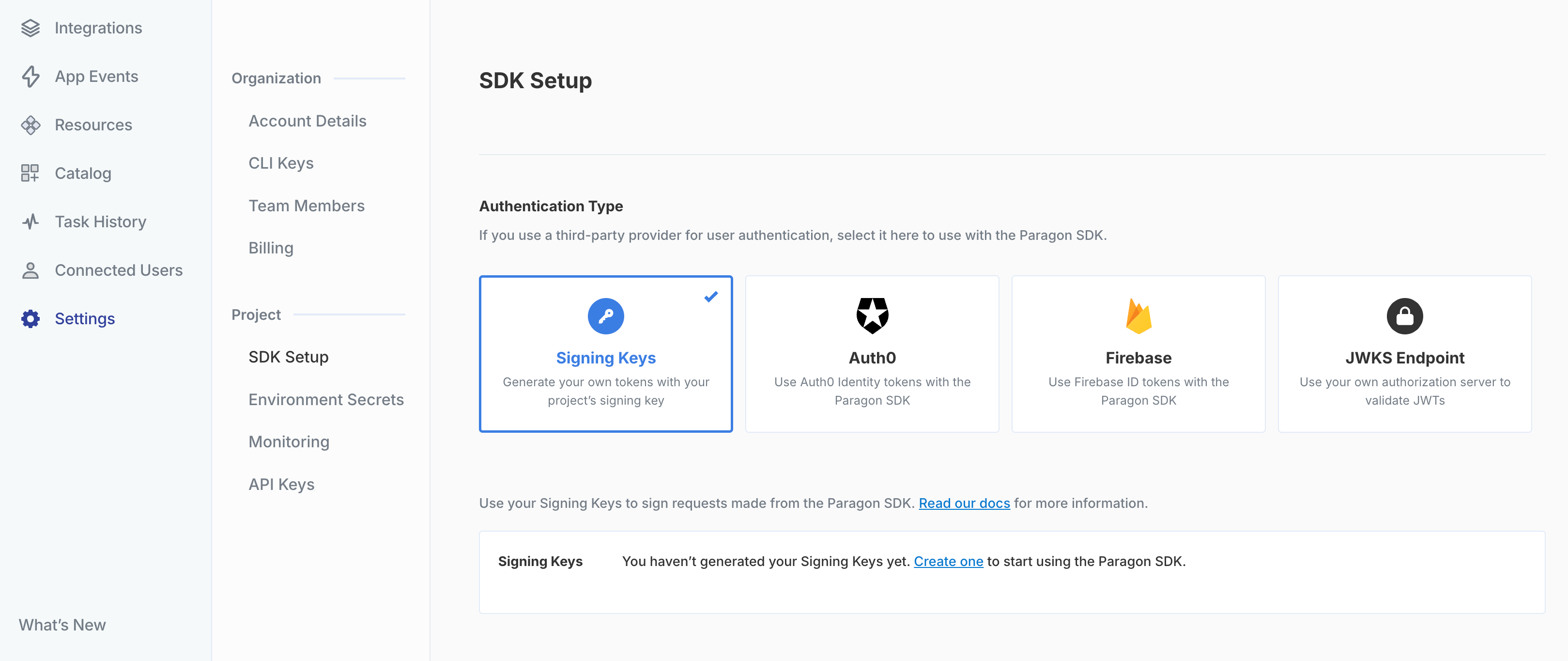
Since Paragon uses a JSON Web Token (JWT) to encode and validate user identity, many managed services will already have a token that you can pass directly to Paragon.
You’ll need to provide Paragon with your Auth0 Tenant Domain, which ends with .auth0.com
. Example: https://<YOUR_TENANT>.auth0.com
.
If you have a domain alias for your tenant domain, use the domain alias instead.
The Auth0 ID token can be used directly as the Paragon User Token.
Auth0 provides comprehensive docs on retrieving the ID token in various contexts. An example of this, using their single page app SDK:
You’ll need to provide Paragon with your Auth0 Tenant Domain, which ends with .auth0.com
. Example: https://<YOUR_TENANT>.auth0.com
.
If you have a domain alias for your tenant domain, use the domain alias instead.
The Auth0 ID token can be used directly as the Paragon User Token.
Auth0 provides comprehensive docs on retrieving the ID token in various contexts. An example of this, using their single page app SDK:
You’ll need to provide Paragon with your Firebase Project ID, which you can find in the Firebase Console.
The Firebase ID token can be used directly as the Paragon User Token.
You can get a Firebase ID token from the JavaScript client-side library with:
You’ll need to provide Paragon with your JWKS Endpoint, which can be found in your identity provider’s documentation.
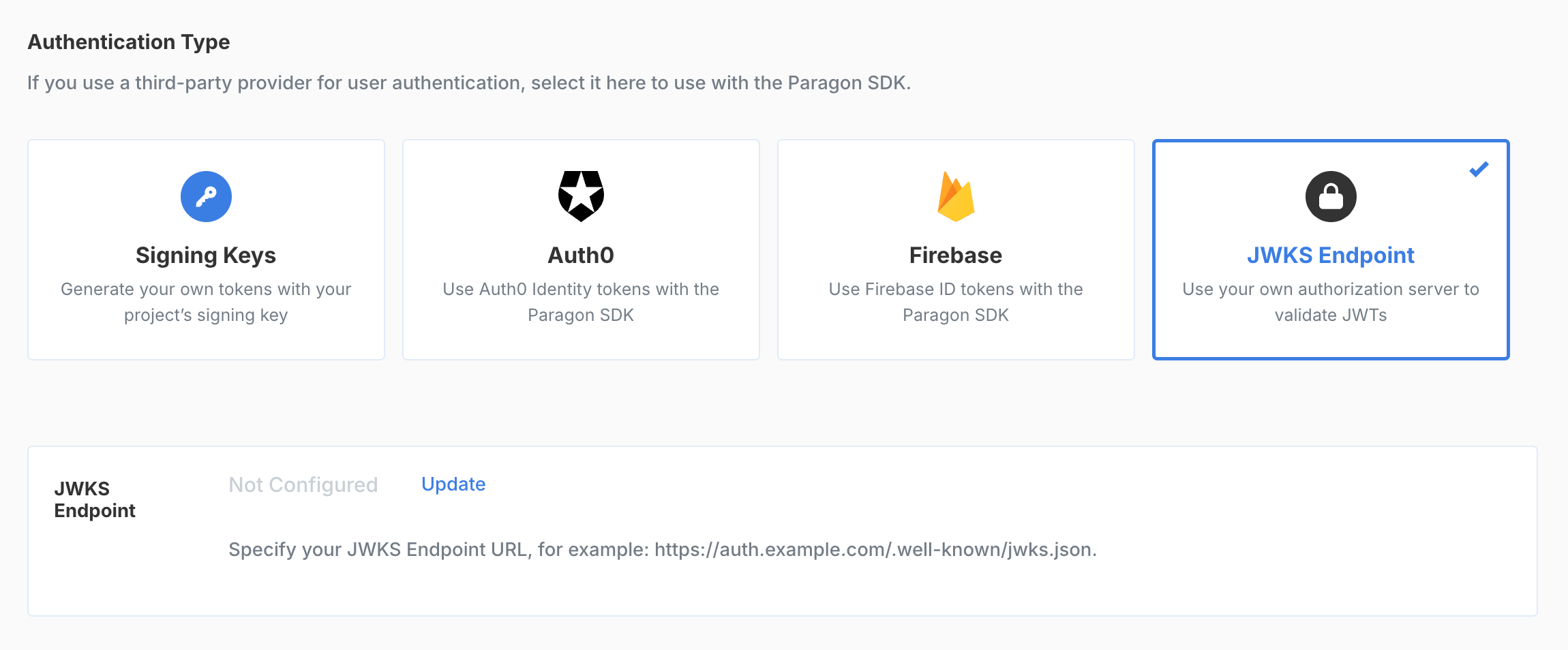
This endpoint will generally have a URL like:
Example: Clerk
To set up Clerk as an authentication provider:
-
Create a blank JWT Template in the Clerk dashboard.
-
Set the Token Lifetime to at least 1 hour (3600 seconds). You will need to call
paragon.authenticate()
with a valid token at least once per period of your token’s lifetime. -
Copy the JWKS Endpoint and add it to the Paragon dashboard.
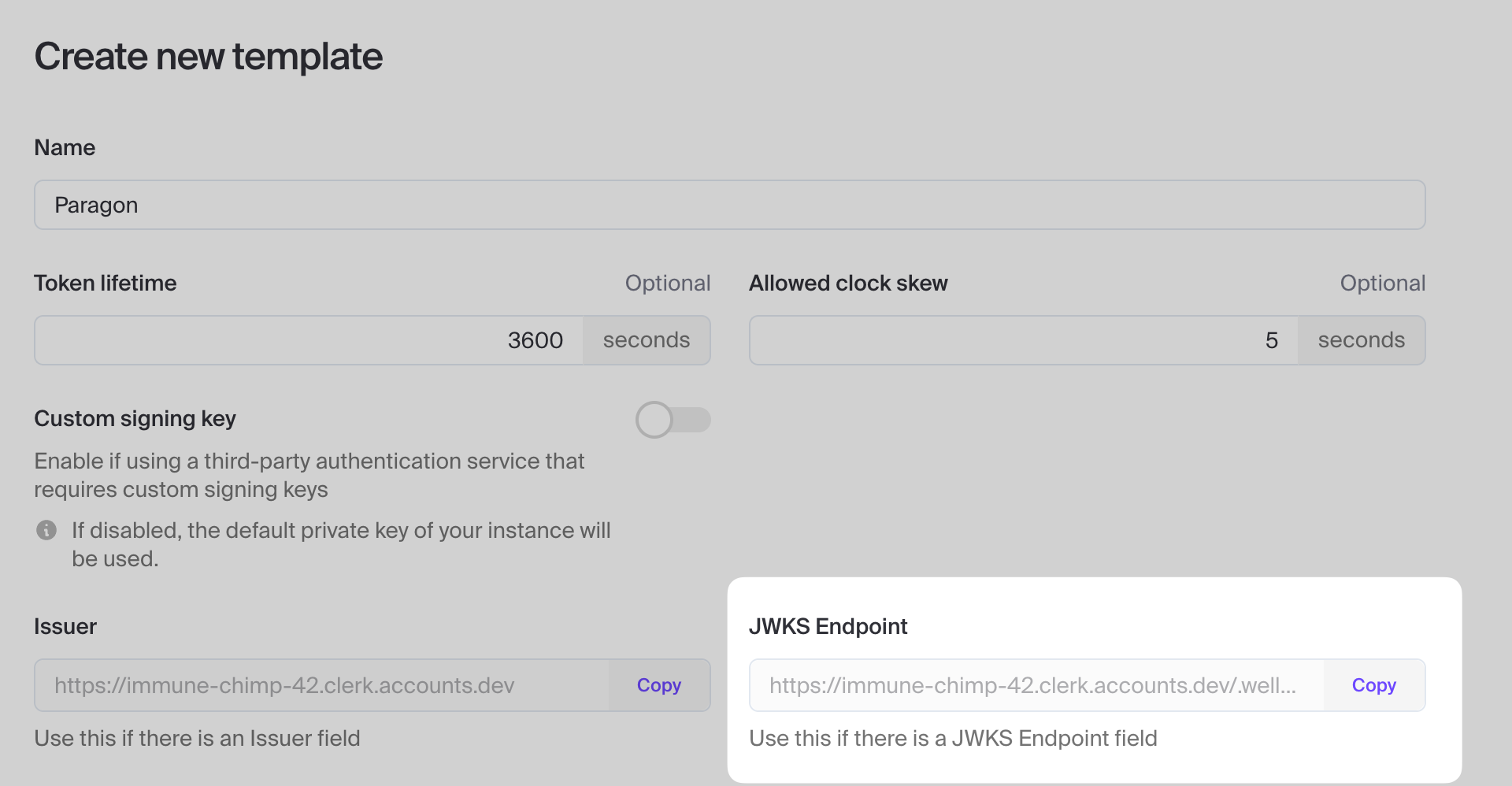
- Call
getToken()
with your template name and pass it to theparagon.authenticate
method: