Paragon
Embed in-app SaaS integrations that connect with your customers’ apps.
Modern businesses expect your B2B/AI SaaS application to integrate seamlessly with their existing tools. Your users may want to:
-
Have your product ingest their external data/permissions for RAG (ie. Google Drive, Notion, Slack, etc.)
-
Maintain a bidirectional sync between your product and their systems of records (ie. CRMs, ERPs, ticketing etc.)
-
Enable your AI agents to interact with their third-party systems
-
Enable pre-built, cross-platform workflows such as Slack notifications or other IFTTT integration logic
However, building and maintaining these native integrations in-house presents significant engineering challenges. Teams must handle authentication, token refresh, API monitoring, rate limiting, and multi-tenant architecture - all while maintaining security and reliability.
Paragon helps developers ship every native integrations 7x faster, letting you focus on your core product instead of integration infrastructure.
New to Paragon? Start a free 14-day trial of Paragon here.
Platform Overview
Core Platform
Benefits you’ll get with every integration you build on Paragon:
-
White-labeled & embedded Connect Portal for your users to enable and configure integration settings
-
Fully managed authentication across all integrations
-
Built-in monitoring and observability
-
Flexible deployment: cloud or on-premise
Get started building Integrations by installing the SDK →
Integration Products
Purpose-built products to implement your desired integration use case.
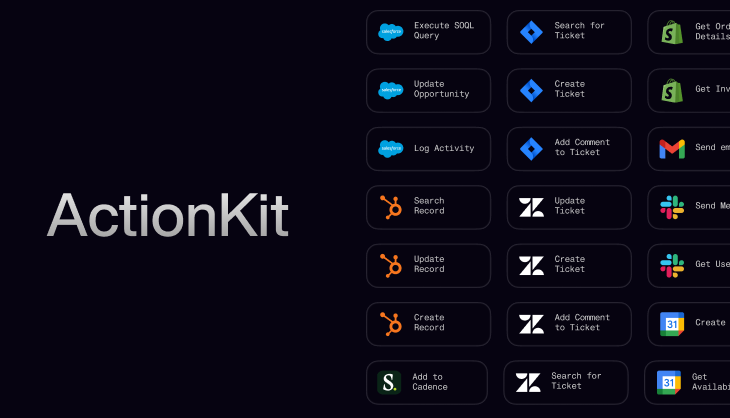
ActionKit
One API to instantly give your AI agent 1000+ integration tools/actions, including:
- Gmail: Send Email
- Google Calendar: Get Calendar Availability
- Salesforce: Create Contact
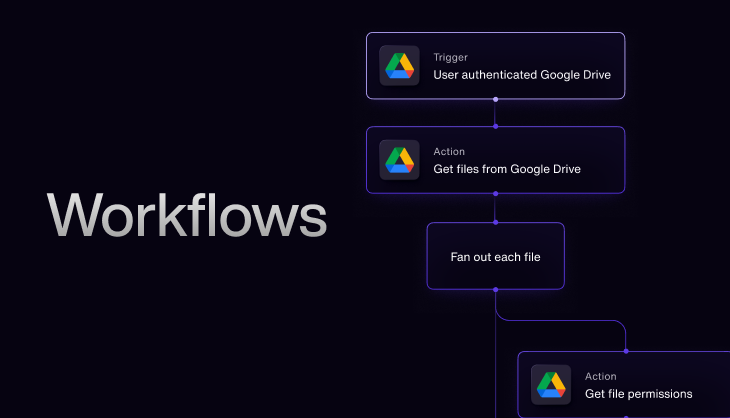
Workflows
Build event-based asynchronous jobs for orchestrating integration logic, such as:
- Data ingestion pipelines (e.g. ingest files from Google Drive or ingest full CRM schema)
- Bidirectional sync (e.g. when a new ticket is created in Jira, sync it to your application)
- Building complex automations (e.g. IFTTT logic)
Was this page helpful?